Here a simple Behavioral coding of the Bubble Sort algorithm is given. This code is written in such a way that it can support any number of inputs with any size. This code is a basic version of the Bubble sort algorithm and sorts the given array in one clock cycle. The code is written for five number of elements.
module bubble_sort #( parameter NUM_VALS = 5, parameter SIZE = 16 )( input wire clk, input wire [NUM_VALS*SIZE-1:0] in, output reg [NUM_VALS*SIZE-1:0] out ); reg [NUM_VALS*SIZE-1:0] sorted_bus; always @(posedge clk) begin out <= sorted_bus; end integer i, j; reg [SIZE-1:0] temp; reg [SIZE-1:0] array [1:NUM_VALS]; always @* begin for (i = 0; i < NUM_VALS; i = i + 1) begin array[i+1] = in[i*SIZE +: SIZE]; end for (i = NUM_VALS; i > 0; i = i - 1) begin for (j = 1 ; j < i; j = j + 1) begin if (array[j] < array[j + 1]) begin temp = array[j]; array[j] = array[j + 1]; array[j + 1] = temp; end end end for (i = 0; i < NUM_VALS; i = i + 1) begin sorted_bus[i*SIZE +: SIZE] = array[i+1]; end end endmodule
The test bench for this code is attached below……….
module sort_tb; reg clk; reg [16-1:0] in1, in2, in3, in4, in5; wire [16-1:0] out1, out2, out3, out4, out5; bubble_sort #(.NUM_VALS(5), .SIZE(16)) dut ( .clk(clk), .in ({in1, in2, in3, in4, in5}), .out({out1, out2, out3, out4, out5}) ); always @(posedge clk) begin in1 <=random; in3 <=
random; in5 <=
display("In: %0d %0d %0d %0d %0d", in1, in2, in3, in4, in5);
finish; end always begin clk = 1'b0; #5; clk = 1'b1; #5; end endmodule
Author Description
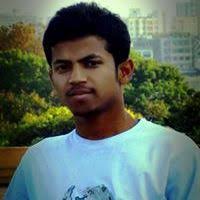
Ardhendu Sarkar
Research Scholar
Computer Science Deptt.
IIEST, Shibpur
email: [email protected]
Ph: 9733242529