Vivado HLS is designed for high level language synthesis of digital systems. Any system can be modelled using lower-level languages like Verilog HDL, VHDL. But these HDL languages are considered as machine level languages like assembly language in case of microprocessors. High level languages like C, C++ or even python also can be used to model the digital systems. The objective of this blog is to discuss few combinational blocks using C or C++ on Vivado HLS platform. This blog will give students basic idea on how to implement basic blocks.
Adder/Subtracter Block
Here, a library that is used is arbitrary length integer. Here, we can choose different length of integers. First code is the main code, and the second code is of the test bench for verification.
#include<ap_int.h>
ap_uint<17> Add_sub(ap_uint<17> inA, ap_uint<17> inB, ap_uint<1> op){
if(op==1)
return( inA + inB);
else
return( inA - inB);
}
#include <stdio.h>
#include <ap_int.h>
ap_uint<17> Add_sub(ap_uint<17> inA, ap_uint<17> inB, ap_uint<1> op);
int main(){
ap_uint<17> inA = 18;
ap_uint<17> inB = 9;
ap_uint<1> op = 1;
printf("\n the result of add_actual is %d", (unsigned int) inA + (unsigned int) inB);
printf("\n the result of sub_actual is %d", (unsigned int) inA - (unsigned int) inB);
ap_uint<17> add, sub;
add = Add_sub(inA,inB,1);
sub = Add_sub(inA,inB,0);
printf("\n the result of add is %d", (unsigned int) add);
printf("\n the result of sub is %d", (unsigned int) sub);
return 0;
}
Simulation of addition and summation of two numbers 18 and 9 is shown below.
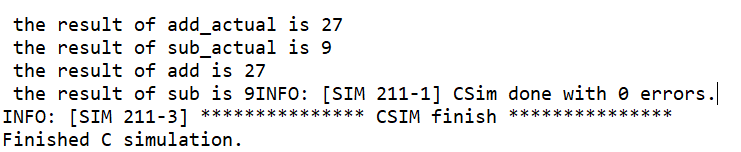
N-bit 2:1 Multiplexer
#include<ap_int.h>
ap_uint<17> muxN_2_1(ap_uint<17> inA, ap_uint<17> inB, ap_uint<1> s){
if(s==0)
return(inA);
else
return(inB);
}
#include <stdio.h>
#include <ap_int.h>
ap_uint<17> muxN_2_1(ap_uint<17> inA, ap_uint<17> inB, ap_uint<1> s);
int main(){
ap_uint<17> inA = 18;
ap_uint<17> inB = 9;
ap_uint<17> op;
op = muxN_2_1(inA,inB,0);
printf("\n Output of mux is %d", (unsigned int) op);
op = muxN_2_1(inA,inB,1);
printf("\n Output of mux is %d", (unsigned int) op);
return 0;
}
N-bit 1:4 Multiplexer
#include<ap_int.h>
ap_uint<17> muxN_4_1(ap_uint<17> inA, ap_uint<17> inB, ap_uint<17> inC,
ap_uint<17> inD, ap_uint<2> s){
if(s==0)
return(inA);
else if (s==1)
return(inB);
else if (s==2)
return(inC);
else if (s==3)
return(inD);
}
#include <stdio.h>
#include <ap_int.h>
ap_uint<17> muxN_4_1(ap_uint<17> inA, ap_uint<17> inB, ap_uint<17> inC,
ap_uint<17> inD, ap_uint<2> s);
int main(){
ap_uint<17> inA = 18;
ap_uint<17> inB = 19;
ap_uint<17> inC = 20;
ap_uint<17> inD = 21;
ap_uint<17> op;
op = muxN_4_1(inA,inB,inC,inD,0);
printf("\n Output of mux is %d", (unsigned int) op);
op = muxN_4_1(inA,inB,inC,inD,1);
printf("\n Output of mux is %d", (unsigned int) op);
op = muxN_4_1(inA,inB,inC,inD,2);
printf("\n Output of mux is %d", (unsigned int) op);
op = muxN_4_1(inA,inB,inC,inD,3);
printf("\n Output of mux is %d", (unsigned int) op);
return 0;
}
2:4 Decoder
#include<ap_int.h>
ap_uint<4> dec2_4( ap_uint<1> en, ap_uint<2> s){
if(en==1)
if (s == 0)
return (1);
else if (s == 1)
return (2);
else if (s == 2)
return (4);
else
return (8);
else
return (1);
}
#include <stdio.h>
#include <ap_int.h>
ap_uint<4> dec2_4( ap_uint<1> en, ap_uint<2> s);
int main(){
ap_uint<4> op;
ap_uint<2> s;
ap_uint<1> en;
op = dec2_4(1,0);
printf("\n Output of decoder is %d", (unsigned int) op);
op = dec2_4(1,1);
printf("\n Output of decoder is %d", (unsigned int) op);
op = dec2_4(1,2);
printf("\n Output of decoder is %d", (unsigned int) op);
op = dec2_4(1,3);
printf("\n Output of decoder is %d", (unsigned int) op);
return 0;
}
Fixed Multiplier
#include<ap_int.h>
ap_uint<17> multN(ap_uint<17> inA, ap_uint<17> inB){
return(inA*inB);
}
#include <stdio.h>
#include <ap_int.h>
ap_uint<17> multN(ap_uint<17> inA, ap_uint<17> inB);
int main(){
ap_uint<17> inA = 18;
ap_uint<17> inB = 9;
ap_uint<17> op;
op = multN(inA,inB);
printf("\n Output of multiplication is %d", (unsigned int) op);
return 0;
}